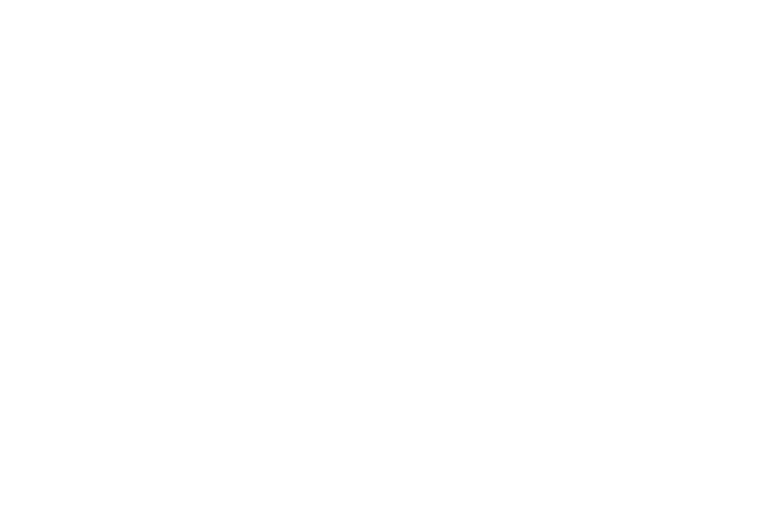
Working with Emoji Id
The EmojiID
struct in the
tari_wallet
crate provides everything you need to work with Tariβs Emoji ID. In this
tutorial, we will learn how to create an Emoji ID from a public key and vice versa. We will also validate an emoji ID
against transcription errors.
use tari_wallet::util::emoji::EmojiId;
use tari_crypto::tari_utilities::hex::Hex;
fn main() {
const EMOJI: &str = "ππ΄π·ππ»ππ©πΎππ¬π§ππ¦π³πππ’πππΈπΏπππππΉππ¬π‘π³π¦πΉπ";
const EMOJI_SHORT: &str = "ππ΄π·ππ»ππ©πΎππ¬π§ππ¦π³πππ’πππΈπΏπππππΉππ¬π‘π³π¦πΉ";
// Convert a public key into its emoji ID
let eid = EmojiId::from_hex("70350e09c474809209824c6e6888707b7dd09959aa227343b5106382b856f73a").unwrap();
println!("{}",eid);
// Convert an emoji to public key (in hex)
let pubkey = EmojiId::str_to_pubkey(EMOJI).unwrap().to_hex();
println!("{}", pubkey);
//Test if both constants declared at the top are valid
assert!(EmojiId::is_valid(EMOJI));
assert_eq!(EmojiId::is_valid(EMOJI_SHORT), false, "Missing checksum");
// TODO - check that emoji ID protects against transcription errors
println!("It's all good!");
}
ππ₯΄πππ¦π€π€ππππ±πππ€³ππβπβπππΆπ€π³π’ππΊππ©π€¬πΌππ₯Ί
70350e09c474809209824c6e6888707b7dd09959aa227343b5106382b856f73a
Itβs all good!